Improve confidence in your code with tests. I'll show you how to use unit, integration, system, and acceptance tests to help you write better code and reduce regression bugs.
This course has a strong focus on web application and REST API testing using Python. We'll work with existing projects and add tests to them using the Python unittest library.
Throughout the course we'll cover mocking and patching of third party dependencies, as well as using Postman for API testing and collaboration.
To write acceptance tests we'll use Behaviour Driven Development and Selenium WebDriver to automate user interactions with a website.
So whether you are an advanced developer looking to get into testing, or a manual tester looking to get into automation, this course will help you understand all the different kinds of tests and how to write them.
Write unit tests using the unittest library
Learn about unit, integration, system, and acceptance testing
Write complete system tests using Python and tools like Postman
Browser-based acceptance testing using Behave and Selenium WebDriver
Set up a continuous integration pipeline using Travis CI
Welcome
3 LESSONS
A Full Python Refresher
47 LESSONS
Introduction to this section
Variables in Python
Coding Exercise 1: variables
String formatting in Python
Getting user input
Writing our first Python app
Lists, tuples, and sets
Advanced set operations
Coding Exercise 2: lists, tuples and sets
Booleans in Python
If statements
The 'in' keyword in Python
If statements with the 'in' keyword
Loops in Python
Coding Exercise 3: flow control-loops and ifs
List comprehensions in Python
Dictionaries
Destructuring variables
Functions in Python
Function arguments and parameters
Default parameter values
Functions returning values
Coding Exercise 4: functions
Lambda functions in Python
Dictionary comprehensions
Coding Exercise 5: dictionaries and students
Unpacking arguments
Unpacking keyword arguments
Object-Oriented Programming in Python
Magic methods: str and repr
Coding Exercise 6: classes and objects
@classmethod and @staticmethod
Coding Exercise 7: @classmethod and @staticmethod
Class inheritance
Class composition
Type hinting in Python 3.5+
Imports in Python
Relative imports in Python
Errors in Python
Custom error classes
First-class functions
Simple decorators in Python
The 'at' syntax for decorators
Decorating functions with parameters
Decorators with parameters
Mutability in Python
Mutable default parameters (and why they're a bad idea)
Your first automated software test
13 LESSONS
Access the code for this section here
Setting up our project
Important: the naming of test files
Writing our first test
Testing dictionary equivalence
Writing blog tests and PyCharm run configurations
The repr method, and intro to TDD
Integration tests and finishing the blog
Mocking, patching, and system tests
Patching the input method and returning values
Taking our patching further
The last few patches!
The TestCase setUp method
Testing a Flask Endpoint
4 LESSONS
REST API Testing, Part I
4 LESSONS
REST API Testing, Part II
4 LESSONS
System testing a REST API
9 LESSONS
Setting project up and creating User model
Change to the next lecture's code
Allowing users to log in
Writing our User tests
The setUpClass method in the BaseTest
Testing user registration
Finalising user System tests
Writing Store System tests
Writing our Item System tests and testing authentication
System testing with Postman and Newman
7 LESSONS
Continuous Integration with Travis CI
9 LESSONS
Acceptance testing and browser automation with Selenium
16 LESSONS
What is acceptance testing?
Introduction to our project
Our first acceptance test step
Getting the Chrome webdriver
Verifying everything works
Finishing our first test
Re-using steps with the regular expression matcher
Our first content test
Page locators and models
The blog page
Using pages in navigation
Don't over-generalise tests!
Waits and timeouts with Selenium
Debugging acceptance tests in PyCharm
Our final complex scenario
Filling in forms with Selenium
5 More Sections
Probably the absolute best class I've ever found on the subject. Sticks with the stock 'unittest'; great explanations of when, why, and how to break up different kinds of tests; and heavy focus on mocking instead of just a gloss over. Excellent teaching. I learned a heck of a lot on this one.
”- Brian Chandler
Awesome course material - very easily understood due to the fantastic training style and knowledge of the instructor. This is great stuff!
”- Theron Lesesne
Very good subtitles, editing, and pacing. Excellent course.
”- William Nguyen
Automated testing is a super power! This is by far the best "I didn't knew this existed" moment in my life.
”- Jorge Ricardo Escobar Carrasco
10/10
”- Grzegorz Werpachowski
Clear, well done and precise, could have had more lectures and hours on Selenium, but other than that, it is an excellent course.
”- Ramy Ahmed Salaheldin Mohamed Anwar Elsaraf
Jose is a wonderful instructor! Thank you!
”- Vladimir Lezgovko
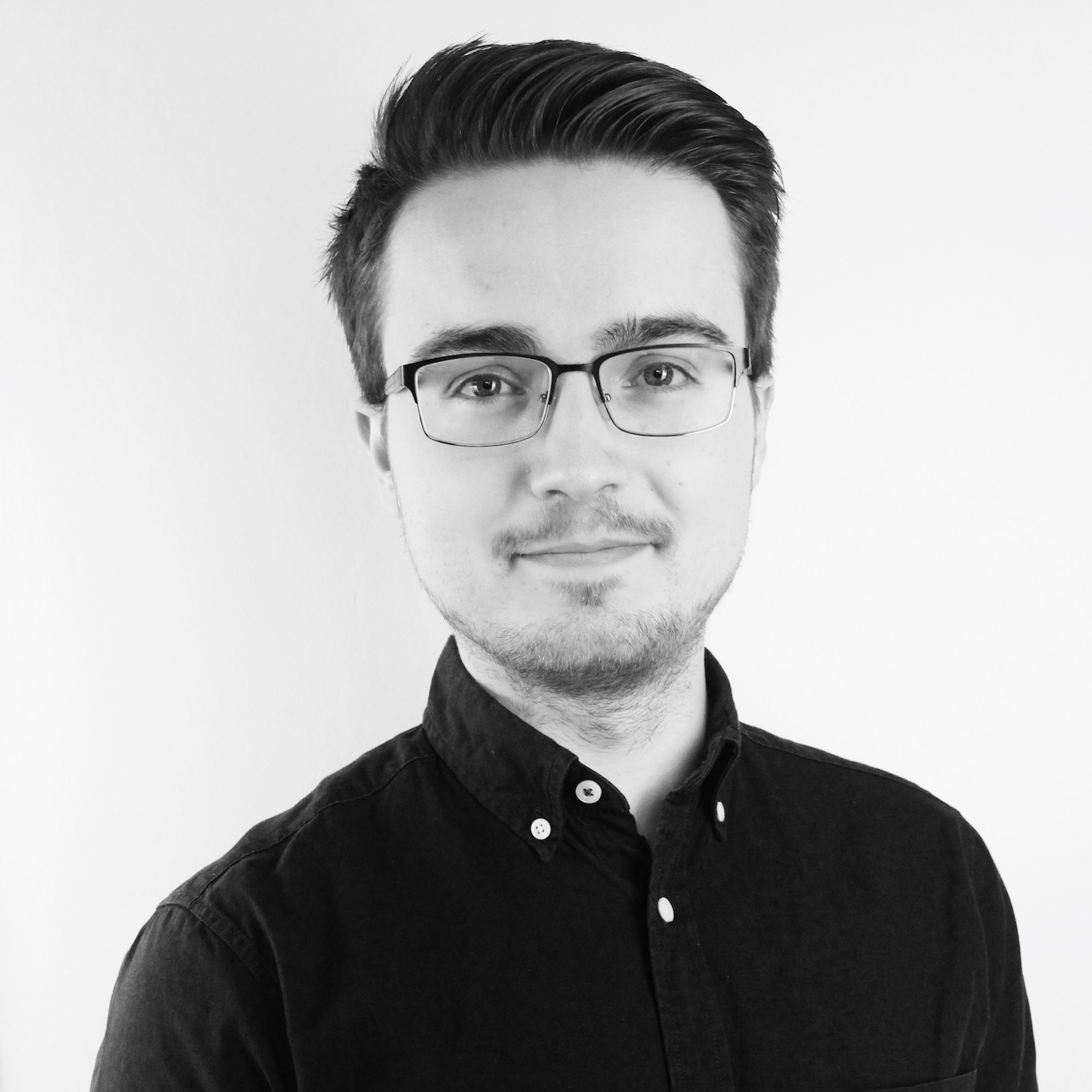
Jose Salvatierra
Hi, I'm Jose! I love helping students learn to code and master software development. I've been teaching online for over 7 years, and I founded Teclado to bring software development to everyone—my objective is for you to truly understand everything that goes on behind the scenes.
How does the course work?
Do I get lifetime access if I buy the course?
Is this course suitable for beginners?
What support is available while taking the course?
Is this course available on Udemy? How is it different from buying it here?